In Swindon at the moment there is this brilliant “game” called “Beat the Street” intended to get people out and about and walking and cycling. They have put more than 170 boxes around the town, strapped to lampposts, and they have given out thousands of fobs and cards.
The way the “game” works is that you tap a box with your fob/card, and then for each subsequent box you tap (within an hour of the previous box) you get 10 points added. Players can be part of a team, so the school, fitness clubs, and businesses of the town are all competing for prizes – in and some cases the competition is hot!!!
It’s been amazing to see loads of people out and about playing – and exercising! I will surely write more blogs about both the social and technical aspects of Beat the Street in the near future.
But I’ve been thinking: what will we do when this ends in late October? I might miss having to check in at the boxes. Is there some way we can “reset” the points and continue the game?
Let’s be clear – the physicality is a huge part of what has made this a success. For kids, being able to have a key-ring like fob in their posession, and being able to tap it on a physical box, and have that box light up and flash in recognition really makes the game. And, the engagement on social media from the Beat the Street team has been brilliant (and I applaud everything they have done here).
BUT…equally, anyone with a tech-head (like me) knows that the vast majority of people tapping will have these smart, connected, location-aware devices with them as they travel around.
So the natural thinking progression is: could we create a virtual version of the game?
And for me, the question is: how hard are the basics here? Especially with the mobile device part. And how much fun can we have finding out.
So today I just tinkered.
The basic premise
No, I’ve not fully thought this through. But on the front end at least, the idea is that you could have a mobile-friendly website with a big button on it. You have a stored list of virtual “box” locations. And when you approach a location and stand within a few metres of it, the button turns green and activates, and you can “check in”.
Notes before we begin:
- Yes, this app has been built a zillion times before. But never by ME! So let me have some fun, will you?
- This is all vanilla JavaScript with no libraries. It’s messy and hacked together in half an hour. Of course it can be better! I’m just tinkering!
Getting location
Getting location in the browser is pretty easy. You need HTTPS to do it, but with that on you just follow the pretty simple Geolocation API and do:
navigator.geolocation.getCurrentPosition(geoSuccess, geoError);
where geoSuccess
and geoError
are functions that take a location parameter. Or you can use:
navigator.geolocation.watchPosition(geoSuccess, geoError);
to watch the position and fire the callback when the position changes.
Simple.
A button
Then you need a button. This is easy to make. I won’t bore you with the fine details of HTML and CSS (because if you’re reading this it’s likely you know them anyway), but I just used:
<button>This is a button</button>
And then
<style>
button {
font-size: 32px;
background-color: #ffbdbd /* Pale red */
padding: 1rem 2rem;
border: 0 none;
outline: none;
border-radius: 8px;
}
button.active {
background-color: #ccffda; /* Pale green */
}
</style>
Showing the location
To help with finding coordinates of “boxes” and generally for figuring this stuff out, I decided I wanted the current location to show. So I added a container for the location result:
<div id="result">
</div>
and then just created the geoSuccess
function, which gets called when the location is found, and have it put the location in this container:
var result = document.getElementById('result');
function geoSuccess(position) {
result.innerHTML='You are at ' +
position.coords.latitude.toFixed(9) +
', ' + position.coords.longitude.toFixed(9);
}
Turning the button green/how big IS the earth?
OK. Now the “fun” bit. We need to work out if we are close to a “box”. But how close should we be?
We could try to work out if were within a circle around the “box” point. But that involves more maths than I want to do right now. Let’s go with an estimate of just “within X metres east/west and X metres north/south”. This, I THINK, means you have to be within a square box around the “box”.
Co-ordinates are given in…what…degrees I guess? So how big is a degree?
Wikipedia says that the earth’s circumference is 40,075.017km around the equator (40,075,017m) and 40007.86km around the poles (40,007,860m).
If we divide by 360, we get 111319.49167m per degree of longitude (east/west) and 111132.94444m per degree of latitude (north/south).
Let’s make things easy and say we’re happy with an 11m box around our “box” – that’s how close you have to be to be able to “check in”. So 11m is about 0.0001 degrees in both directions. I’m sure someone will point out something about elevation and the curvature of the earth, but it’s good enough for now.
Rather than start with a list of boxes, lets just start with one location that activates the button. So all I need is some latitude and longitude I want to get close enough to in order to activate the button. Once I have this I can update my geoSuccess
function:
var boxLong = <some co-ordinate>;
var boxLat = <some co-ordinate>;
var result = document.getElementById('result');
var button = document.querySelector('button');
function geoSuccess(position) {
result.innerHTML='You are at ' +
position.coords.latitude.toFixed(9) +
', ' + position.coords.longitude.toFixed(9);
if (Math.abs(boxLong - position.coords.longitude) < 0.0001 &&
Math.abs(boxLat - position.coords.latitude) < 0.0001) {
button.classList.add('active');
} else {
button.classList.remove('active');
}
}
Now, of course, this is just one coordinate. In reality you’re going to want to loop or map over a load of coordinates and find if you’re close to any of them. But this is the basics of a working system.
I’ve taken these basics and added some better styling and added a little to them to make a simple “app”. You can walk along and place a box in your current location using a button. The “check in” button is then green until you’re 11m (0.0001 degrees!) away when it turns red. It turns green again when you back inside the 11m range.
Here it is in action (with annotations):
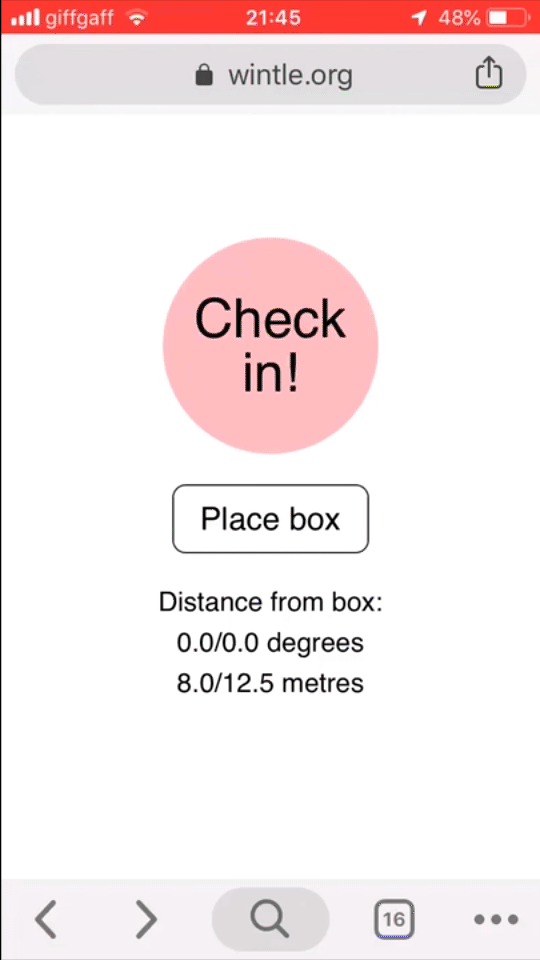
OK. So this is just a bit of fun. But it’s the basis of something quite interesting: you could do a virtual beat-the-street style game using people’s smartphones to check in to virtual boxes.
You can have a play at: https://zapthemap.com/ (It’s evolved a lot since this post!)
YES, yes, yes. This is (relatively) trivial to hack by simulating your location in a web browser. You wouldn’t want to run any kind of genuinely competitive real game using it. But it could form the basis of some friendly competition or some kind of personal game with yourself. I dunno.
But it was fun, and surprisingly easy, to get this far.