I used some hacky JavaScript to scrape my friends birthdays from Facebook so that I can put them in my actual calendar.
This is a bit technical and probably not something you want to do. And it may not even work for you. And it’s probably not allowed. But I’d argue that if you can see all your friends birthdays on Facebook anyway then this is just an automated version of something you could do manually.
But anyway, I wanted to record what I did.
So here’s the situation: I’m not really on Facebook. But there are some people I’m friends with on there for whom I wanted reminders of their birthdays. So I wanted to be able to download just these dates so that I can save them for my own use.
The first step is to open your browser’s dev tools, and go to the Facebook Birthdays page: https://www.facebook.com/friends/birthdays
On the network tab you’ll now see all the network requests.
Hit Ctrl/Cmd-F to open the find window and search for friends_by_birthday_month_context_sentence
This will show all the requests that contain the birthday data. It will look something like this:
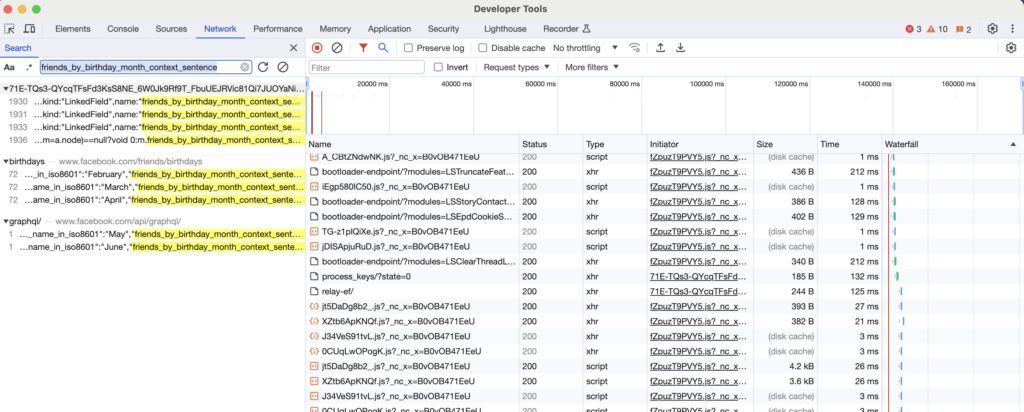
On the left, in the search results, you can see the /birthdays
request with results for February, March and April. (Note: I’m doing this in January!) And then a follow-up request to /graphql
that gets the data for May and June.
If you scroll the page down the other months load as well, but you’ll need to reload the search to get the full results.
You then need to get the data from these requests into somewhere you can run JavaScript. And this will need some manual effort.
Processing the /birthdays request
For the /birthdays
request you’ll need to track down the script that contains friends_by_birthday_month_context_sentence
Then copy that script into a code editor (I actually did this in an interactive JavaScript tool called RunJS). Do NOT use an online code editor like CodePen or JSBin as this file contains the personal data of your friends!!!
Then edit the code to assign the JSON from the response to a variable by prepending it with
const data =
At the end of this file you should then add code to extract the names and dates. Mine looks like this:
let friends_by_months = data.require[0][3][0]['__bbox'].require[5][3][1]['__bbox'].result.data.viewer.all_friends_by_birthday_month.edges.flatMap(m => m.node.friends.edges)
friends_by_months.map(f => f.node.name + ',' + f.node.birthdate.year + '-' + f.node.birthdate.month + '-' + f.node.birthdate.day)
Yes. This is a mess. But if you run this JS code it you should get out an array of names and birthdays for the first few months.
Processing the /graphql requests
The requests for the other months are GraphQL requests. You’ll need to do something similar to what you did for the main birthdays
request for each of the GraphQL requests containing birthday data.
Copy the JSON response into a code editor and prepend with:
const data =
The code to extract the dates is slightly different. But putting this at the end of the file and executing it should give you the array of names and dates:
let friends_by_months = data.data.viewer.all_friends_by_birthday_month.edges.flatMap(m => m.node.friends.edges)
friends_by_months.map(f => f.node.name + ',' + f.node.birthdate.year + '-' + f.node.birthdate.month + '-' + f.node.birthdate.day)
That’s all I got! Maybe this is useful to someone.